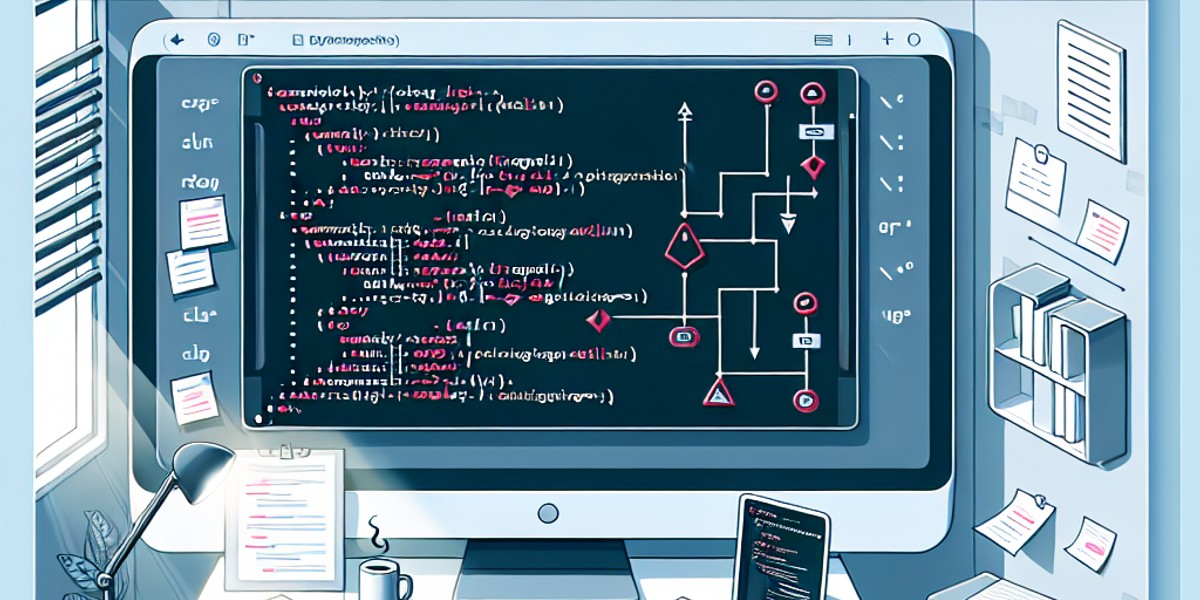
Ruby Function Argument Types
Ruby Function Arguments Programming
Introduction
Ruby is a versatile and expressive programming language that provides various ways to pass arguments to functions (or methods). These argument types play a crucial role in method design and functionality. In this article, we will explore the different types of arguments in Ruby functions, providing detailed examples for each type, and then compare the pros and cons of each approach.
I. Positional Arguments
Positional arguments are the most basic and commonly used type of arguments in Ruby functions. They are defined in the method signature and must be provided in the order specified when calling the method. Let’s look at an example:
def add_numbers(a, b)
result = a + b
return result
end
sum = add_numbers(5, 3)
puts "The sum is #{sum}" # Outputs: The sum is 8
In this example, a
and b
are positional arguments, and they are required. You must provide values for them in the same order they are defined in the method signature.
Pros of Positional Arguments:
- Simplicity: They are straightforward to use and understand.
- Order matters: You can control the order of arguments, which can be useful for clarity in some cases.
Cons of Positional Arguments:
- Lack of Flexibility: If you need to omit or change the order of arguments, you may need to modify the method signature.
II. Default Arguments
Default arguments, also known as optional arguments, allow you to provide default values for parameters in the method definition. If the caller does not provide a value for an optional argument, the default value is used. Here’s an example:
def greet(name, greeting = "Hello")
puts "#{greeting}, #{name}!"
end
greet("Alice") # Outputs: Hello, Alice!
greet("Bob", "Hi") # Outputs: Hi, Bob!
In this example, greeting
is an optional argument with a default value of “Hello.” If the caller provides a value for greeting
, it overrides the default value.
Pros of Default Arguments:
- Flexibility: You can make parameters optional, providing sensible default values.
- Backward Compatibility: You can add optional arguments to existing methods without breaking existing code.
Cons of Default Arguments:
- Limited to the end: Ruby requires default arguments to come after required arguments in the method signature.
III. Keyword Arguments
Keyword arguments allow you to pass arguments to a function by specifying the parameter names along with their values explicitly. This approach enhances code readability and can make method calls more self-explanatory. Here’s an example:
def order(product:, quantity:)
puts "Order placed for #{quantity} #{product}(s)."
end
order(product: "Widget", quantity: 5) # Outputs: Order placed for 5 Widget(s).
In this example, product:
and quantity:
are keyword arguments. They are named and can be provided in any order, making the method call more explicit and less error-prone.
Pros of Keyword Arguments:
- Clarity: Method calls are self-documenting, improving code readability.
- Flexibility: You can provide arguments in any order.
- Future-proof: You can add new keyword arguments without affecting existing code.
Cons of Keyword Arguments:
- Slightly more verbose: They require the use of colons and can be longer than positional or default arguments.
IV. Splat Arguments
Splat arguments allow you to pass an arbitrary number of arguments to a method as an array. There are two types of splat arguments: single splat (*args
) and double splat (**kwargs
). Single splat collects additional positional arguments into an array, while double splat collects additional keyword arguments into a hash. Let’s look at examples of both:
Single Splat (Collecting Positional Arguments)
def sum(*numbers)
total = numbers.sum
return total
end
result = sum(1, 2, 3, 4, 5)
puts "The sum is #{result}" # Outputs: The sum is 15
In this example, *numbers
is a single splat argument that collects all provided positional arguments into an array called numbers
.
Pros of Single Splat Arguments:
- Flexibility: You can accept any number of additional arguments.
- Array manipulation: You can easily work with the collected arguments as an array.
Cons of Single Splat Arguments:
- Less explicit: It may be less clear what types of arguments the method expects.
Double Splat (Collecting Keyword Arguments)
def options(**params)
puts "Received options: #{params}"
end
options(first_name: "Alice", last_name: "Johnson", age: 30)
# Outputs: Received options: {:first_name=>"Alice", :last_name=>"Johnson", :age=>30}
In this example, **params
is a double splat argument that collects all provided keyword arguments into a hash called params
.
Pros of Double Splat Arguments:
- Flexibility: You can accept any number of additional keyword arguments.
- Hash manipulation: You can easily work with the collected arguments as a hash.
Cons of Double Splat Arguments:
- Less explicit: It may be less clear what types of arguments the method expects.
V. Mixed Arguments
Ruby allows you to combine different argument types in a single method definition. You can have positional arguments, default arguments, keyword arguments, and splat arguments in the same method. Here’s an example:
def mixed_args(a, b = 10, *numbers, product:, quantity: 1, **options)
puts "a: #{a}, b: #{b}, numbers: #{numbers}, product: #{product}, quantity: #{quantity}, options: #{options}"
end
mixed_args(1, 2, 3, 4, 5, product: "Widget", quantity: 3, color: "blue", size: "large")
In this example, the mixed_args
method accepts a combination of positional arguments, default arguments, keyword arguments, and splat arguments. It demonstrates the flexibility Ruby offers when it comes to designing method signatures.
Certainly! Working with Ruby function arguments can be powerful and flexible, but there are also some tips, tricks, and potential pitfalls to be aware of. Let’s explore some of these:
Tips and Tricks
-
Use Keyword Arguments for Clarity: Whenever possible, use keyword arguments. They make your code more readable and self-documenting, as the argument names are explicitly provided in the method call.
def send_email(subject:, to:, body:) # ... end send_email(subject: "Hello", to: "[email protected]", body: "Hi there!")
-
Provide Default Values Thoughtfully: Default arguments are helpful, but be cautious about setting them too liberally. Overuse of default arguments can lead to unexpected behavior and make your code less predictable.
-
Avoid Mixing Default and Splat Arguments: Be cautious when mixing default and splat arguments, as it can sometimes lead to unexpected results. Ensure that the order of arguments is clear when using both.
-
Use Splat Arguments for Variadic Methods: When you need to accept a variable number of arguments, single splat (
*args
) and double splat (**kwargs
) arguments are your friends. They allow you to work with arbitrary argument lists.def sum(*numbers) numbers.sum end
-
Consider Method Overloading: Ruby does not support method overloading like some other languages, but you can achieve similar functionality by using optional arguments and conditionals within the method.
def calculate(*args) if args.length == 2 # Perform binary operation elsif args.length == 3 # Perform ternary operation else # Handle invalid input end end
Common Pitfalls
-
Changing the Order of Default Arguments: If you need to change the order of default arguments in a method with existing usage, it can break existing code. Be cautious when making such changes and consider versioning or documenting them carefully.
-
Overusing Mixed Arguments: While mixed arguments offer flexibility, overusing them can lead to complex method signatures that are hard to understand. Strive for clarity and readability in your code.
-
Unintended Side Effects with Default Arguments: Default arguments are evaluated once when the method is defined, not every time the method is called. Be aware of this behavior when using mutable objects as default values.
def add_item(item, cart = []) cart << item end cart1 = add_item("apple") cart2 = add_item("banana") puts cart1.inspect # Both cart1 and cart2 are [ "apple", "banana" ]!
-
Inconsistent Naming Conventions: Be consistent with your naming conventions for arguments. If you use underscores (
snake_case
) for one argument, stick with it throughout your codebase. Inconsistent naming can lead to confusion. -
Ignoring Splat Arguments: If you accept splat arguments but don’t use them within the method, it can be a source of confusion for developers using your code. Ensure that you handle splatted arguments appropriately.
-
Losing Information with Double Splat: When using double splat (
**kwargs
), you can lose information about the order in which keyword arguments were provided. This may not be an issue in most cases, but it’s something to keep in mind.
In conclusion, understanding and effectively using Ruby function arguments is essential for writing clean, maintainable code. Use the right argument type for the job, document your methods clearly, and be mindful of potential pitfalls to avoid unexpected behavior in your Ruby applications. Good practices and clear documentation will help you and your team work more efficiently and with fewer surprises in your code.