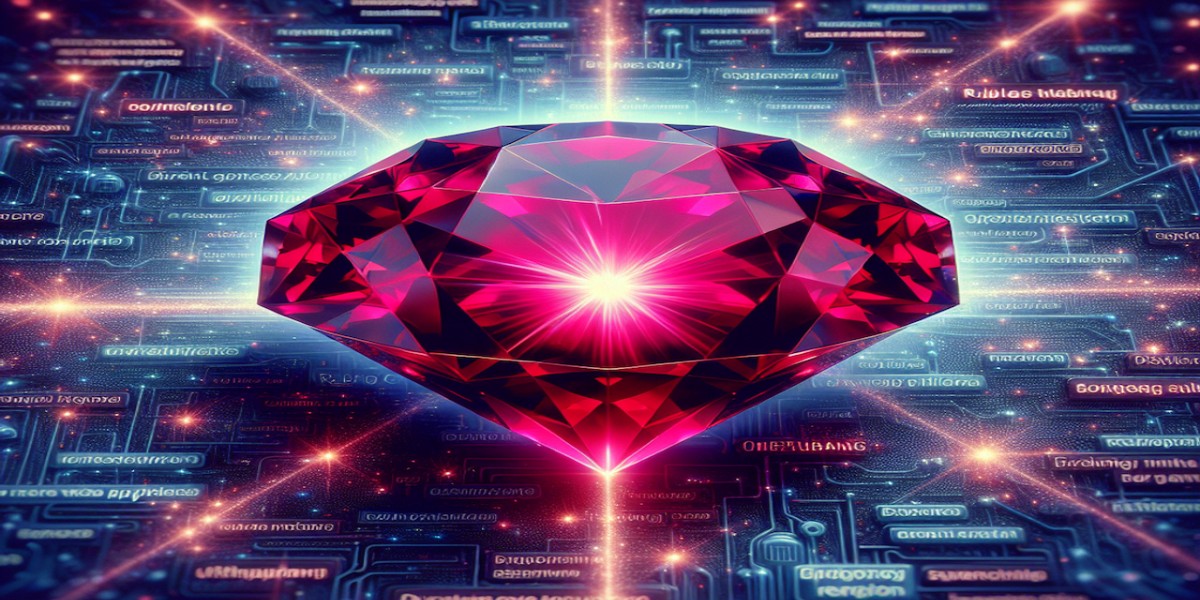
Lesson Plan: Mastering Advanced Concepts in Ruby on Rails
Ruby on Rails Lesson Plan
Objective
By the end of this lesson plan, students will have a deep understanding of advanced concepts in Ruby on Rails, enabling them to develop complex and scalable web applications with confidence.
Duration
This lesson plan is designed to span over several weeks, with each topic covered in one or more sessions.
Prerequisites
- Basic understanding of Ruby programming language
- Familiarity with fundamental concepts of Ruby on Rails framework
- Experience building simple Rails applications
Lesson 1: Metaprogramming in Ruby
Study Materials
- “Metaprogramming Ruby 2: Program Like the Ruby Pros” by Paolo Perrotta
- Online tutorials and resources on metaprogramming in Ruby
Topics to Cover
- Understanding method_missing
- Using define_method and instance_eval
- Creating dynamic methods and attributes
Activities
- Reading and discussing relevant chapters from “Metaprogramming Ruby 2”
- Writing and experimenting with metaprogramming techniques in a sample Rails application
Lesson 2: Advanced ActiveRecord
Study Materials
- Rails Guides: ActiveRecord Associations
- Online tutorials and documentation on ActiveRecord
Topics to Cover
- Polymorphic associations
- ActiveRecord callbacks
- Advanced querying techniques
Activities
- Exploring and implementing polymorphic associations in a sample Rails application
- Writing custom callbacks and understanding their lifecycle
- Practicing advanced querying techniques with ActiveRecord
Lesson 3: Background Jobs and Task Queues
Study Materials - Part 1
- Sidekiq Documentation
- DelayedJob Documentation
- Blog posts and tutorials on background job processing in Rails
Topics to Cover
- Introduction to background job processing
- Setting up Sidekiq or DelayedJob in a Rails application
- Writing and scheduling background jobs
Activities
- Installing and configuring Sidekiq or DelayedJob in a sample Rails application
- Implementing background jobs for time-consuming tasks
Lesson 4: Rails Engines and Modularization
Study Materials
- Rails Guides: Engines
- Online tutorials and blog posts on Rails engines
Topics to Cover
- Introduction to Rails engines
- Creating and mounting engines within a Rails application
- Sharing functionality across multiple applications
Activities
- Building a simple Rails engine to encapsulate reusable functionality
- Integrating the engine into a sample Rails application
Lesson 5: Testing and Test-Driven Development
Study Materials
- “RSpec Rails” by David Chelimsky, Myron Marston, et al.
- RailsGuides: Testing Rails Applications
Topics to Cover
- Test-driven development (TDD) principles
- Writing unit tests, integration tests, and system tests
- Using RSpec or Minitest for testing Rails applications
Activities
- Implementing test coverage for critical features in a sample Rails application
- Practicing TDD by writing tests before implementing new features
Lesson 6: Performance Optimization and Security
Study Materials
- “Rails Performance” by Nate Berkopec
- Rails Security Guides
Topics to Cover
- Performance optimization techniques (e.g., caching, database indexing)
- Security best practices (e.g., CSRF protection, parameter sanitization)
- Using performance monitoring tools (e.g., New Relic, Skylight)
Activities
- Identifying and addressing performance bottlenecks in a sample Rails application
- Implementing security measures and conducting security audits
Assessment
- Periodic quizzes or coding exercises to assess understanding of each topic
- Final project where students apply advanced concepts learned throughout the course to build a complex Rails application
References
- “Metaprogramming Ruby 2: Program Like the Ruby Pros” by Paolo Perrotta
- Rails Guides and Documentation
- Online tutorials, blog posts, and documentation for specific topics
- “RSpec Rails” by David Chelimsky, Myron Marston, et al.
- “Rails Performance” by Nate Berkopec
Lesson 7: Continuous Integration and Deployment
Study Materials
- “Continuous Delivery: Reliable Software Releases through Build, Test, and Deployment Automation” by Jez Humble and David Farley
- Documentation and tutorials on Continuous Integration (CI) and Continuous Deployment (CD) tools like Jenkins, Travis CI, or GitHub Actions
Topics to Cover
- Setting up CI/CD pipelines for Rails applications
- Automating testing, building, and deployment processes
- Ensuring code quality and reliability through automated checks
Activities
- Configuring a CI/CD pipeline for a sample Rails application using a chosen tool
- Automating tests, builds, and deployment processes
Lesson 8: Advanced Frontend Integration
Study Materials
- Rails Guides: Asset Pipeline
- Documentation and tutorials on frontend frameworks like React or Vue.js
Topics to Cover
- Integrating frontend frameworks with Rails applications
- Using webpacker for managing frontend assets
- Building modern, interactive interfaces with Rails and JavaScript
Activities
- Integrating a frontend framework (e.g., React) into a sample Rails application
- Exploring webpacker and setting up asset compilation for JavaScript and CSS
Lesson 9: API Development with Rails
Study Materials
- “Craft GraphQL APIs in Elixir with Absinthe” by Bruce Williams and Ben Wilson
- Rails Guides: API Applications
Topics to Cover
- Building RESTful APIs with Rails
- Introduction to GraphQL and its implementation with Rails
- Authentication and authorization for APIs
Activities
- Developing a RESTful API for a sample Rails application
- Experimenting with GraphQL and implementing a simple GraphQL API
Lesson 10: Advanced Topics and Beyond
Study Materials
- Conference talks, podcasts, and articles on advanced Rails topics
- Open-source Rails projects and community contributions
Topics to Cover
- Exploring advanced Rails features like Action Cable (WebSocket integration), ActiveStorage (file uploads), and Action Mailbox (incoming emails)
- Contributing to open-source Rails projects and engaging with the Rails community
Activities
- Contributing bug fixes or new features to a selected open-source Rails project
- Experimenting with advanced Rails features and integrating them into a sample application
Conclusion and Recap
- Review of key concepts and skills covered throughout the course
- Encouragement to continue learning and exploring advanced Rails topics independently
- Suggestions for further reading, resources, and avenues for continued professional growth
This extended lesson plan aims to provide students with a comprehensive understanding of advanced Ruby on Rails concepts, including topics such as continuous integration, frontend integration, API development, and exploring advanced Rails features. Through a combination of theoretical study, practical exercises, and real-world projects, students will gain the skills and confidence needed to excel as expert developers in the Ruby on Rails ecosystem.