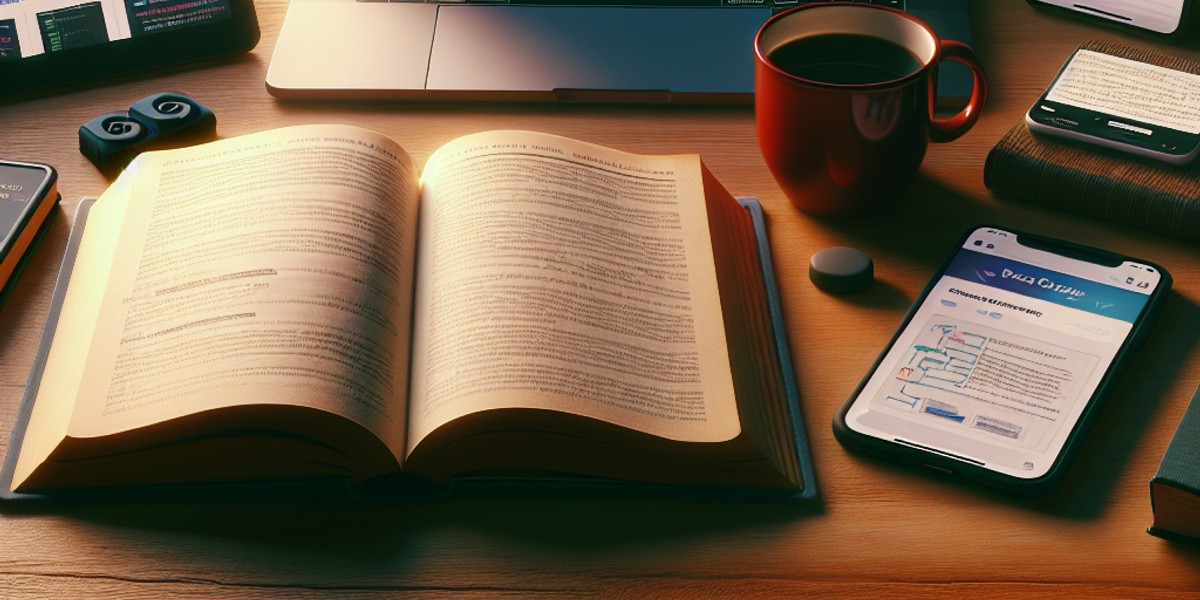
10 Powerful Tips and Tricks for Ruby on Rails Development
Ruby on Rails Web Development Ruby Rails Tips and Tricks
INTRODUCTION
Ruby on Rails is a popular web development framework known for its elegance and productivity. Whether you’re a seasoned Rails developer or just starting out, mastering some tips and tricks can greatly enhance your development workflow and boost the efficiency of your Rails projects. In this blog post, we will explore 10 powerful tips and tricks for Ruby on Rails development that can help you write cleaner code, improve performance, and streamline your development process.
Optimize Database Queries
Efficient database queries are crucial for improving performance. Utilize ActiveRecord query optimization techniques like eager loading (includes
), selective column fetching (pluck
), and query caching to minimize the number of database hits and reduce latency.
Leverage Rails Generators
Rails generators automate repetitive tasks and speed up development. Use rails generate
command to quickly scaffold models, controllers, views, migrations, and more. Customize your own generators to streamline your project-specific patterns and conventions.
Employ Partials and View Helpers
Deduplicate your view code by using partials and view helpers. Partials allow you to extract reusable components, while view helpers encapsulate complex logic into reusable methods, enhancing code reusability and readability.
Implement Caching
Improve the performance of your Rails application by implementing caching techniques. Utilize fragment caching (cache
and render
methods) and low-level caching (Rails.cache
) to cache expensive computations, database queries, and views, resulting in faster response times.
Utilize Background Jobs
Offload time-consuming tasks to background jobs using frameworks like Sidekiq or Delayed Job. Perform tasks asynchronously to enhance user experience, improve scalability, and prevent delays caused by blocking operations.
Apply Model Validations
Ensure data integrity and prevent invalid data from entering the database by implementing model validations. Leverage built-in ActiveRecord validations (presence
, length
, uniqueness
, etc.) or create custom validations to enforce business rules and maintain data consistency.
Employ Concerns and Modules
Organize your codebase and promote code reuse by using concerns and modules. Extract common functionalities into concerns or modules and include them in your models or controllers, reducing duplication and enhancing code maintainability.
Use Strong Parameters
Protect your application from mass assignment vulnerabilities by using strong parameters. Whitelist specific attributes in your controllers using permit
method to ensure only allowed parameters are accepted for mass assignment.
Implement Authentication and Authorization
Secure your application by implementing authentication and authorization mechanisms. Utilize gems like Devise or Authlogic for user authentication and CanCanCan or Pundit for authorization, controlling access to different parts of your application based on user roles and permissions.
Write Tests
Adopt a test-driven development (TDD) or behavior-driven development (BDD) approach to ensure the quality and stability of your Rails application. Write unit tests (RSpec
or Minitest
) and integration tests (Capybara
or RSpec
) to cover critical functionalities and catch regressions.
CONCLUSION
These 10 powerful tips and tricks for Ruby on Rails development provide valuable insights and techniques to optimize your Rails projects. By leveraging these techniques, you can write cleaner code, improve performance, and streamline your development process. Keep exploring the vast Rails ecosystem and stay updated with best practices to continuously enhance your Rails development skills and build robust web applications.
Remember, mastering Rails is an ongoing journey, and practice combined with continuous learning is the key to becoming a proficient Rails developer.